How to Create a Custom Accordion Using HTML, CSS, and jQuery
A jQuery accordion is a user interface element that allows you to show and hide content in a vertical manner. It is a useful component for organizing and displaying a lot of information in a limited space. When one section of the accordion clicks, it expands to reveal its content while the others collapse.
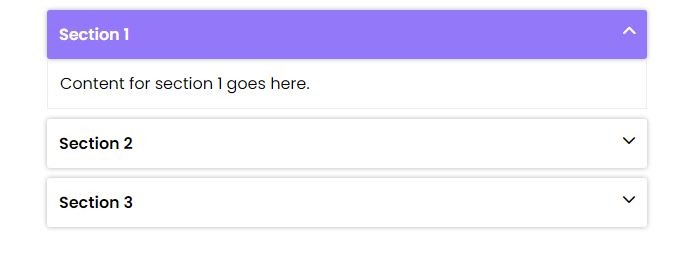
To create a custom jQuery accordion, you can follow these steps:
- Step 1: HTML Structure
- Step 2: CSS Styles
- Step 3: jQuery Code
Step 1: HTML Structure
Create a basic HTML structure for the accordion. The accordion should have a container element with several child elements that represent the accordion sections. Each section should contain a header element and a content element.
<div class="ab_accordion">
<div class="ab_accordion_section">
<div class="ab_accordion_header">Section 1 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content">
Content for section 1 goes here.
</div>
</div>
<div class="ab_accordion_section">
<div class="ab_accordion_header">Section 2 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content">
Content for section 2 goes here.
</div>
</div>
<div class="ab_accordion_section">
<div class="ab_accordion_header">Section 3 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content">
Content for section 3 goes here.
</div>
</div>
</div>
Step 2: CSS Styles
Add CSS styles to hide the content elements by default and style the headers to indicate that they can be clicked.
* {
margin: 0px;
padding: 0px;
}
body {
font-family: 'Poppins', sans-serif;
}
.ab_accordion {
width: 100%;
max-width: 600px;
margin: auto;
}
.ab_accordion_content {
display: none;
}
.ab_accordion_header {
cursor: pointer;
background-color: #ffffff;
padding: 12px;
font-weight: bold;
box-shadow: 0 0 5px rgba(0, 0, 0, .3);
margin-top: 10px;
border-radius: 3px;
}
.ab_accordion_header.active {
background-color: #9379f9;
color: #fff;
}
.ab_accordion_content {
padding: 12px;
animation: fade-in-up 0.3s ease-in-out;
border-left: 1px solid #ededed;
border-right: 1px solid #ededed;
border-bottom: 1px solid #ededed;
}
.ab_accordion_header i {
float: right;
font-size: 14px;
margin-top: 2px;
}
.ab_accordion_header.active i {
transform: rotate(180deg);
}
@keyframes fade-in-up {
0% {
opacity: 0;
transform: translateY(20px);
}
100% {
opacity: 1;
transform: translateY(0);
}
}
Step 3: jQuery Code
Write jQuery code to handle the accordion behavior. When a header is clicked, the corresponding content should be shown and the other contents should be hidden.
<script src="https://code.jquery.com/jquery-3.6.4.min.js"
integrity="sha256-oP6HI9z1XaZNBrJURtCoUT5SUnxFr8s3BzRl+cbzUq8=" crossorigin="anonymous"></script>
<script>
$(document).ready(function () {
$('.ab_accordion_header').click(function () {
// toggle the content
$(this).next('.ab_accordion_content').slideToggle(200);
// toggle the arrow icon
$(this).toggleClass('active');
// hide the other contents
$('.ab_accordion_content').not($(this).next()).slideUp(200);
// remove the active class from other headers
$('.ab_accordion_header').not($(this)).removeClass('active');
});
});
</script>
Additional:
If you want to show by default open first accordion add an active class in insideab_accordion_header and add a display block inside ab_accordion_content HTML as bellow.
<div class="ab_accordion">
<div class="ab_accordion_section">
<div class="ab_accordion_header active">Section 1 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content" style="display:block">
Content for section 1 goes here.
</div>
</div>
<div class="ab_accordion_section">
<div class="ab_accordion_header">Section 2 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content">
Content for section 2 goes here.
</div>
</div>
<div class="ab_accordion_section">
<div class="ab_accordion_header">Section 3 <i class="fas fa-chevron-down"></i></div>
<div class="ab_accordion_content">
Content for section 3 goes here.
</div>
</div>
</div>