How to Create Text Converter Tools using jQuery
Updated on
...
25th September 2023
In this article, we are going to generate text converter tools for this we will use html CSS and Bootstrap to design the basic structure of the form, and jQUery is used to implement the logic to generate text converter with condition and also for the copy button.
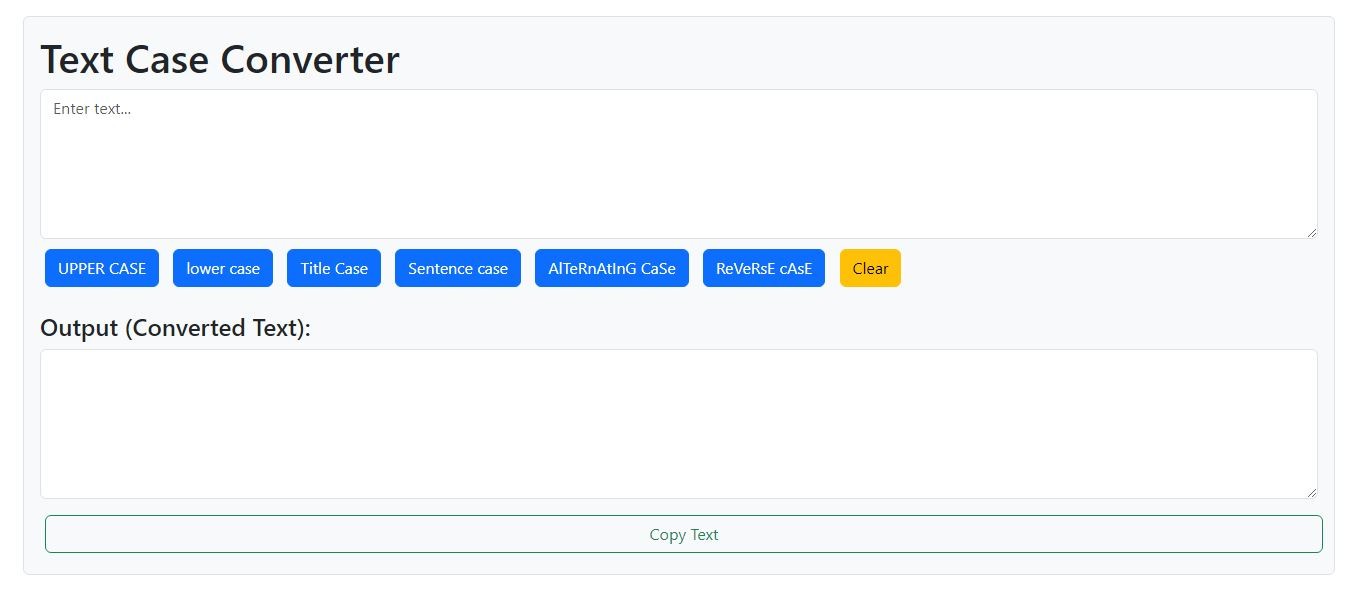
Step To Follow:
- Step 01: Create HTML Structure
- Step 02: Add Jquery CDN and bootstrap CDN
- Step 03: Create Text Converter and copy text jQuery functions
- Step 04: Write Some Custom CSS for Styling
Step 01: Create HTML Structure
<div class="container p-3 text_converter_tool">
<div class="row">
<div class="col-md-12 border p-3 shadow-sm rounded bg-light">
<h1>Text Case Converter</h1>
<textarea id="inputText" placeholder="Enter text..." class="form-control"></textarea>
<div class="options">
<button class="btn btn-primary" id="convertToUpper">UPPER CASE</button>
<button class="btn btn-primary" id="convertToLower">lower case</button>
<button class="btn btn-primary" id="convertToTitle">Title Case</button>
<button class="btn btn-primary" id="convertToSentence">Sentence case</button>
<button class="btn btn-primary" id="convertToAlternating">AlTeRnAtInG CaSe</button>
<button class="btn btn-primary" id="convertToReverse">ReVeRsE cAsE</button>
<button id="clearText" class="btn btn-warning">Clear</button>
</div>
<h4>Output (Converted Text):</h4>
<textarea id="outputText" readonly class="form-control"></textarea>
<button id="copyText" class="btn btn-outline-success w-100 mt-3">Copy Text</button>
</div>
</div>
</div>
Step 02: Add Jquery CDN and bootstrap CDN
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 03: Create a Text Converter and copy Text jQuery functions
$(document).ready(function () {
$("#convertToUpper").click(function () {
var inputText = $("#inputText").val();
$("#outputText").val(inputText.toUpperCase());
});
$("#convertToLower").click(function () {
var inputText = $("#inputText").val();
$("#outputText").val(inputText.toLowerCase());
});
$("#convertToTitle").click(function () {
var inputText = $("#inputText").val();
var words = inputText.split(" ");
for (var i = 0; i < words.length; i++) {
words[i] = words[i].charAt(0).toUpperCase() + words[i].slice(1).toLowerCase();
}
$("#outputText").val(words.join(" "));
});
$("#convertToSentence").click(function () {
var inputText = $("#inputText").val();
var sentences = inputText.split(". ");
for (var i = 0; i < sentences.length; i++) {
sentences[i] = sentences[i].charAt(0).toUpperCase() + sentences[i].slice(1).toLowerCase();
}
$("#outputText").val(sentences.join(". "));
});
$("#convertToAlternating").click(function () {
var inputText = $("#inputText").val();
var alternatingText = "";
for (var i = 0; i < inputText.length; i++) {
var char = inputText.charAt(i);
alternatingText += i % 2 === 0 ? char.toUpperCase() : char.toLowerCase();
}
$("#outputText").val(alternatingText);
});
// $("#convertToReverse").click(function () {
// var inputText = $("#inputText").val();
// var reversedText = inputText.split("").reverse().join("");
// $("#outputText").val(reversedText);
// });
$("#convertToReverse").click(function () {
var inputText = $("#inputText").val();
var reversedCaseText = "";
for (var i = 0; i < inputText.length; i++) {
var char = inputText.charAt(i);
if (char === char.toUpperCase()) {
reversedCaseText += char.toLowerCase();
} else {
reversedCaseText += char.toUpperCase();
}
}
$("#outputText").val(reversedCaseText);
});
$("#copyText").click(function () {
var outputText = $("#outputText");
outputText.select();
document.execCommand('copy');
// Change button text and then revert it after a delay
$("#copyText").text("Copied");
setTimeout(function () {
$("#copyText").text("Copy Text");
}, 1000);
});
$("#clearText").click(function () {
$("#inputText").val(""); // Clear input text
$("#outputText").val(""); // Clear output text
});
});
Step 04: Write Some Custom Style
.text_converter_tool {
margin: 0 auto;
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0px 0px 5px rgba(0, 0, 0, 0.2);
}
.text_converter_tool textarea {
min-height: 150px !important;
}
.text_converter_tool .options {
text-align: left;
margin-top: 5px;
margin-bottom: 20px;
}
.text_converter_tool button {
margin: 5px;
padding: 5px 10px;
border: none;
background-color: #007bff;
color: #fff;
cursor: pointer;
border-radius: 5px;
}
.text_converter_tool button:hover {
background-color: #0056b3;
}