How to Create SLUG or URL Generator Tools using jQuery
Updated on
...
18th September 2023
In this article, we will use html css and bootstrap to design the basic structure of the form, and jQUery is used to implement the logic to generate slug with condition and aslo for the copy button.
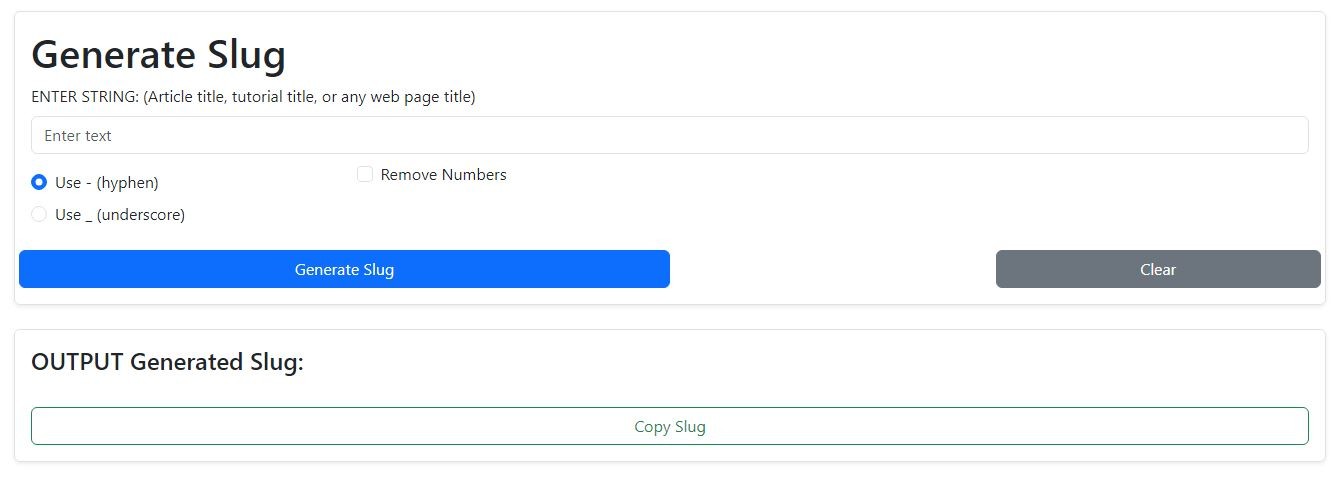
Step To Follow:
- Step 01: Create HTML Structure
- Step 02: Add Jquery CDN and bootstrap CDN
- Step 03: Create Slug Generator and copy slug functions
Step 01: Create HTML Structure
<div class="container p-3">
<div class="row">
<div class="col-md-12 border p-3 shadow-sm rounded">
<h1>Generate Slug</h1>
<div class="form-group my-2">
<label for="slug_input">ENTER STRING: (Article title, tutorial title, or any web page title)</label>
<input type="text" id="slug_input" placeholder="Enter text" class="form-control mt-2">
</div>
<div class="row">
<div class="col-3">
<div class="form-check my-2">
<input class="form-check-input" type="radio" name="slug_rule" id="slug_rule1" checked
value="-">
<label class="form-check-label" for="slug_rule1">Use - (hyphen)</label>
</div>
<div class="form-check my-2">
<input class="form-check-input" type="radio" name="slug_rule" id="slug_rule2" value="_">
<label class="form-check-label" for="slug_rule2">Use _ (underscore)</label>
</div>
</div>
<div class="col-6">
<div class="form-check">
<input class="form-check-input" type="checkbox" id="removeNumbers">
<label class="form-check-label" for="removeNumbers">Remove Numbers</label>
</div>
</div>
</div>
<div class="row d-flex justify-content-between pt-3">
<button id="generateSlugButton" class="btn btn-primary w-50">Generate Slug</button>
<button id="clearButton" class="btn btn-secondary w-25">Clear</button>
</div>
</div>
<div class="col-md-12 border p-3 shadow-sm mt-4 rounded">
<h4 class="mb-3">OUTPUT Generated Slug:</h4>
<h3> <span id="slugOutput"></span></h3>
<button id="copyButton" class="btn btn-outline-success w-100 mt-3">Copy Slug</button>
</div>
</div>
</div>
Step 03: Create a Slug Generator and copy slug functions
function generateSlug(inputText, separator, removeNumbers) {
var slug = inputText
.toLowerCase()
.replace(/\s+/g, separator)
.replace(/[^\w\-]+/g, '')
.replace(/^-+/, '')
.replace(/-+$/, '');
if (removeNumbers) {
slug = slug.replace(/\d+/g, ''); // Remove numbers
}
slug = slug.replace(new RegExp(separator + '+', 'g'), separator); // Remove consecutive separators
return slug;
}
$(document).ready(function () {
$('#generateSlugButton').click(function () {
var inputText = $('#slug_input').val();
var separator = $('input[name="slug_rule"]:checked').val();
var removeNumbers = $('#removeNumbers').is(':checked');
var slug = generateSlug(inputText, separator, removeNumbers);
$('#slugOutput').text(slug);
});
$('#clearButton').click(function () {
$('#slug_input').val('');
$('#slugOutput').text('');
});
$('#copyButton').click(function () {
var slug = $('#slugOutput').text();
if (slug) {
var tempInput = document.createElement('input');
tempInput.value = slug;
document.body.appendChild(tempInput);
tempInput.select();
document.execCommand('copy');
document.body.removeChild(tempInput);
$('#copyButton').html("Copied")
// alert('Slug copied to clipboard: ' + slug);
setTimeout(function () {
$('#copyButton').html('Copy Slug');
}, 1500); // 2000 milliseconds = 2 seconds
}
});
});
Step 02: Add Jquery CDN and bootstrap CDN
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>